Today we're going to take a look at how to quickly get started with the Indiegogo API. In this short tutorial we'll be showcasing a simple implementation that will allow you to pull some data from your Indiegogo campaign and display it on your website. You can make use of this tutorial with basic working knowledge of HTML and CSS. If you'd like to take things further and have some Javascript knowledge as well this example should be a great starting point to building out your own basic integration that showcases your campaign data on your site!
Building the HTML
For this example we want to showcase some campaign stats on a web page. We're going to display how much the campaign has raised and how many people have contributed to it. In the code below we set up two boxes that will hold these numbers once we call them from the API. Copy this code into the body of your HTML.
<div class="igg-wrapper">
<div class="igg-boxes" id="igg-funds">
<h3>Raised so far</h3>
</div>
<div class="igg-boxes" id="igg-contributions">
<h3>People have contributed</h3>
</div>
</div>
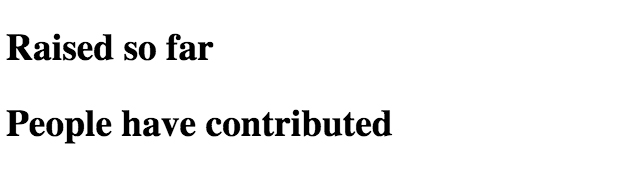
Great! We've now got a place to show our stats, but as you can see above - it's not pretty. Let's add some really basic styling to it using CSS. Open up your CSS file and copy the code below into it.
.igg-wrapper {
height: 300px;
width: 900px;
margin: 0 auto;
}
.igg-boxes {
height: 90%;
width: 290px;
float: left;
background-color: #2E2E1F;
margin: 5px 5px 5px 5px;
opacity: 0.8;
border-radius: 30px;
}
.igg-boxes h2 {
color: #FFFFFF;
opacity: 1.0;
text-align: center;
font-size: 35px;
}
.igg-boxes h3 {
color: #FFFFFF;
opacity: 1.0;
text-align: center;
font-size: 25px;
}
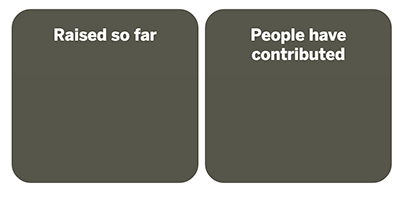
That's a little better! You'll of course want to change the styling to fit with your site design, but for this example all we need is the basics. Now that that's out of the way, let's move on to the good part and start pulling some data.
Grabbing the Data
Here's where all the work is done. We're going to put some javascript into our HTML that will make a call using the API to get the data we need to populate the boxes we built up above. The first thing we need to do is set the page up to use the JQuery library. Paste the code below into the of your HTML file. We'll be using JQuery to build up the javascript function that's going to get us our data.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
For the sake of simplicity, we're going to put our javascript into the HTML as well. One thing to note is that anything you write into the HTML is going to be easily viewable by anyone - this includes your api_token. You'll typically want to hide your api_token but that's outside the scope of this example. If you end up building something out based off of this, don't ever expose your access_token in the HTML!
The code below makes a call the contributions endpoint of the API for a specified campaign. It then takes that data and looks for the collected_funds and goal. Those three responses are then inserted into the DOM using JQuery to place the numbers where we want them. You'll also see a function at the end of the code that adds commas to our results to make them a bit more readable.
Paste the code below into the of your HTML. Be sure to replace the [YOUR API TOKEN] and [YOUR CAMPAIGN ID] with your corresponding info!
<script type="text/javascript" language="javascript">
$(document).ready(function() {
$.getJSON("https://api.indiegogo.com/1/campaigns/[YOUR CAMPAIGN ID].json?api_token=[YOUR API TOKEN]", function(gogodata) {
$("#igg-funds").prepend('<h2>$' + numberWithCommas(gogodata.response.collected_funds) + '<br> out of <br>$' + numberWithCommas(gogodata.response.goal) + '</h2>');
$("#igg-contributions").prepend('<h2>' + numberWithCommas(gogodata.response.contributions_count) + '</h2>');
});
});
function numberWithCommas(x) {
return x.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
}
</script>
And that's it! You now have a working example of how to pull your campaign data using the Indiegogo API and show it on your own website!
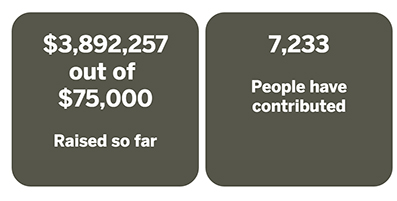